Reporting Data APIs
Querying Data
There are two types of read queries on the Reporting Service: aggregation and search. Any Reporting Object Configuration can be used either for an aggregation or a paginated search, however in practice collections are designed to work either for aggregation (chart display) or paginated search (paginated list).
Reporting Search Expressions
As in all search use cases throughout memority, Querying Reporting data is performed using Search Expressions. This section details the specifics of their use in Reporting queries.
Search Properties
For a given Reporting Object Configuration, one may search on all of its declared criteria that are not of type ANY
or ANY_ARRAY
.
A dotted path, e.g. identity.id
denotes a path in a Document, and not an object reference as in other Search Expression use cases.
Available operators
The following operators are available:
EQUALS
NOT_EQUALS
LIKE
NOT_LIKE
EQUALS_LIKE
NOT_EQUALS_LIKE
STARTS_WITH_LIKE
ENDS_WITH_LIKE
CONTAINS_LIKE
STARTS_WITH
ENDS_WITH
CONTAINS
GREATER
LESS
GREATER_OR_EQUALS
LESS_OR_EQUALS
BETWEEN
IN
NOT_IN
IS_NOT_NULL
IS_NULL
IN_LAST
IN_NEXT
IN_THE_PAST
IN_THE_FUTURE
Their semantics is same as usual, except for “fuzzy” (*LIKE
) operators: they are case insensitive, but not diacritics-insensitive.
Matching on Objects
To perform an exact match on an OBJECT
criterion, use the EQUALS
operator with a JSON value:
_id = '{"id": "902d54d28d090217", "date": "1970-01-01T00:00:00Z"}'
The JSON value object will be pre-processed recursively according to the declared criteria to apply type conversions and correct key order.
Matching on arrays
For arrays of simple values (STRING_ARRAY
, INT_ARRAY
,…), the standard Search Expression Operator behavior regarding multivalued properties applies: an Operator Expression on an _ARRAY
criterion will match all Documents where the array contains at least one value matching the constraint. For instance, someArray > 42
will match all Documents where someArray
contains at least one value greater than 42.
The same behavior applies to the EQUALS
operator on OBJECT_ARRAY
criteria: ids = '{"id": "902d54d28d090217", "date": "1970-01-01T00:00:00Z"}'
will match all documents where ids
contains the specified value.
To search on object arrays (either explicit OBJECT_ARRAY
or implicit ANY_ARRAY
with sub-criteria), use the containsElementMatching
Search Expression Function accepting the following arguments:
Mandatory | Description | Example | |
---|---|---|---|
1 | YES | A simple |
|
2 | YES | The constraint on the array elements, without the array path prefix. Can be any Search Expression, and may contain nested |
|
Example :
containsElementMatching(
path = 'actors.candidates',
firstName = 'John' AND lastName = 'Doe'
)
Will match all Documents where actors.candidates
contains at least one element matching firstName = 'John' AND lastName = 'Doe'
.
REST API
Operation | Method | URL | Request |
---|---|---|---|
Aggregation | POST | api/rep/collections/{reportingObjectConfigurationId}/~aggregate |
JSON
The above request will
Each groups wille be returned as a Document with a single |
Search | POST | api/rep/collections/{reportingObjectConfigurationId}/~search |
JSON
|
Response
Depending on the type of requests, the result will be a fully aggregated result or a paginated result.
Access rights
A right for execution on the specific configuration id is required.
Execution right |
---|
|
Inserting or updating data
General architecture
Write operations are asynchronous: there is no guarantee that the write operation has already been effectively performed upon API call return.
To write in a Collection the sys.rep-collection-write
system right must be granted to the caller.
Furthermore, write operations can only be performed through configurations with the property collectionWriteConfiguration.writable
set to true.
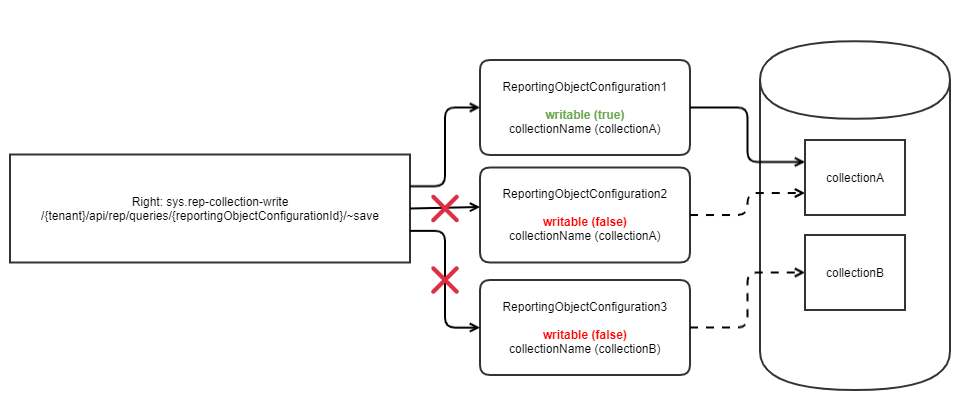
REST API
Operation | Method | URL | Request |
---|---|---|---|
Documents insertion or update | POST | api/rep/collections/{reportingObjectConfigurationId}/~save | Asynchronously inserts a list of documents in the Collection targeted by this Reporting Object Configuration id. The The insertion of documents is done using an unordered strategy, meaning that order of documents in the supplied list can be different after execution. If there is a problem with the insertion of a document, this strategy will also ignore the problematic document and continue the bulk insertion for remaining documents. Provided that the A maximum or 10 000 Documents can be inserted in a single request. Example of payload:
CODE
|
Response
HTTP 200 with no body since write operations are asynchronous (documents modifications are queued on an AMQP exchange).
Access right
A system right for writing in collections is required.
Execution right |
---|
sys.rep-collection-write |
Groovy API
An API_REPORTING scripting API is exposed in all rules to insert and update documents from the Identity Management, Business Management and Synchronization services:
// Insert a new document
API_REPORTING.on("reporting-object-configuration-id-1")
.document()
.field("a", 1)
.field("b", 2)
.end()
.save()
// Insert a document (map) already defined
API_REPORTING.on("reporting-object-configuration-id-1")
.document(map)
.save()
// Insert a list of documents
API_REPORTING.on("reporting-object-configuration-id-1")
.documents(listsOfDocuments)
.save()
// Combination of the different previous examples
API_REPORTING.on("reporting-object-configuration-id-1")
.documents([ // list of documents
[fieldOne: 1, fieldTwo: "one"],
[fieldOne: 2, fieldTwo: "two"]
])
.document([fieldOne: 3, fieldTwo: "three"]) // single document
.document() // document builder
.field("fieldOne", 4)
.field("fieldTwo", "four")
.end()
.save()